- CodeCraft by Dr. Christine Lee
- Posts
- APIs: Your Gateway to Cool Connections!💻🤝💻
APIs: Your Gateway to Cool Connections!💻🤝💻
A Beginner’s Guide

TL;DR
APIs (Application Programming Interfaces) enable different software systems to communicate with each other.
They act as digital messengers, exchanging data and services between applications.
In this post, we’ll explain APIs with fun illustrations, break down technical terms, and show you how to use the ChatGPT API in Python with a step-by-step code example.
What is an API?
Imagine you're at a restaurant.
Instead of cooking the food yourself, you give your order to a waiter (the API), who takes it to the kitchen (the server) and returns with your dish.
In this analogy:
You: The user or client making a request.
API (Waiter): The messenger that takes your request to the server and delivers the response.
Server (Kitchen): Processes the request and sends back the result.
Illustration:
User ↔️ API (Waiter) ↔️ Server (Kitchen)
Why Are APIs Important?
APIs are critical because they:
Simplify development: Instead of building everything from scratch, developers reuse services (e.g., Google Maps API for location data).
Enable integrations: Apps can talk to each other, like connecting payment gateways or weather data to mobile apps.
Enhance automation: APIs allow seamless data flow between systems without human intervention.
Key API Terms Explained for Beginners
Here are some key terms you’ll encounter:
Endpoint: A specific URL where the API provides a service (like
https://api.openai.com/v1/chat/completions
).Request: A message sent to the API asking for data or action.
Response: The data the API sends back, usually in JSON format (a lightweight data exchange format).
{ "user": "Alexa", "message": "Hello!" }
API Key: A unique identifier that authorizes your access to the API.
If you enjoyed learning how to use APIs with Python, take it a step further by exploring today’s sponsor - Pinata's File API.
With it, you can store and manage files securely using IPFS or private storage, bringing Web3 capabilities to your Python projects.
Learn more about Pinata's powerful file management solutions.
Streamline your development process with Pinata’s easy File API
Easy file uploads and retrieval in minutes
No complex setup or infrastructure needed
Focus on building, not configurations
Using the ChatGPT API with Python: A Step-by-Step Example
Here’s a simple Python example that sends a message to ChatGPT using its API and prints the response.
Step 1: Install Required Library
Open your terminal or command prompt and type:
pip install openai
Step 2: Set Up Your API Key
Create an account on OpenAI.
Generate an API key from your OpenAI dashboard.
Store the key securely (we’ll hard-code it here for simplicity, but use environment variables for real projects).
Step 3: Write the Python Code
Here’s the code to call the ChatGPT API and print the response:
import openai
# Step 1: Set up your API key
openai.api_key = "your-api-key-here"
# Step 2: Define the message you want to send
prompt = "Explain how an API works in simple terms."
# Step 3: Send the request to the API
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[{"role": "user", "content": prompt}]
)
# Step 4: Extract and print the API's response
message = response.choices[0].message['content']
print(message)

Python Code on PyCharm
Before Running the Code
Make sure the openai
library version is compatible.
Enter this command in the terminal:
pip install openai==0.28
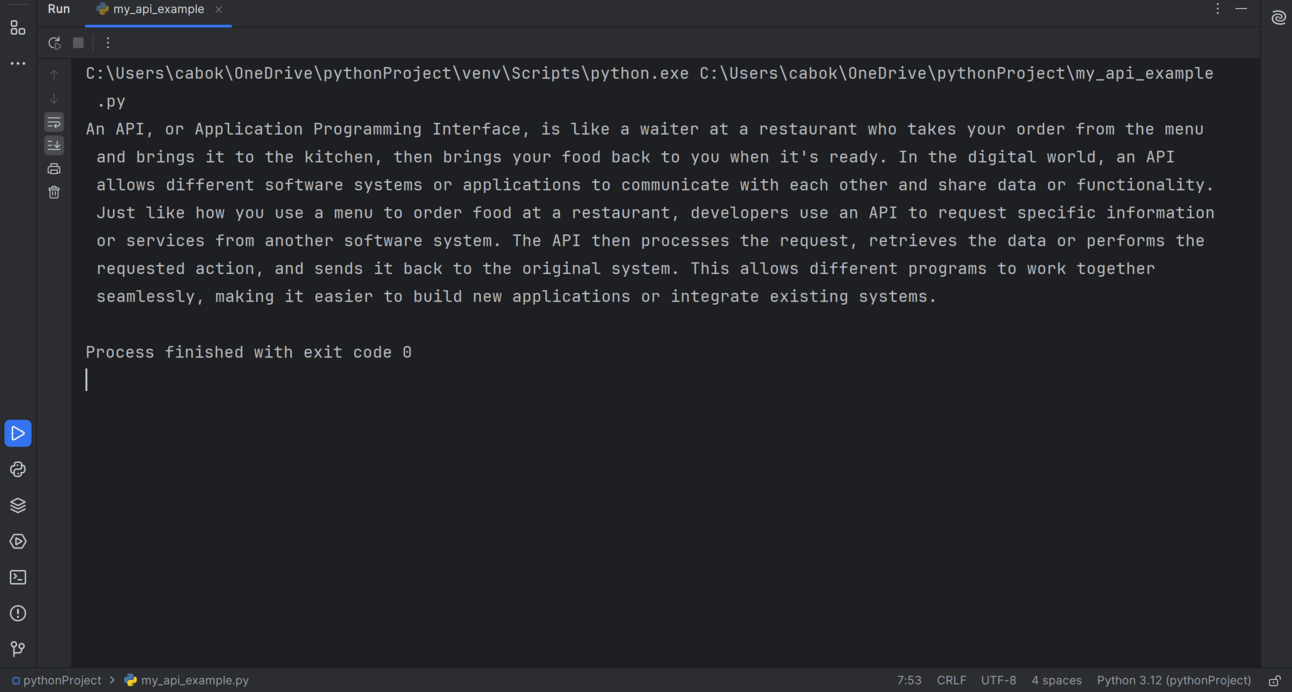
Output from the Python Code
Step 4: Explanation of the Code
Import the library: The
openai
library helps us interact with the ChatGPT API.Set the API key: This authorizes your request to use the service.
Create a prompt: This is what you want ChatGPT to answer.
Send the request: We use
ChatCompletion.create()
to send the message to the API.Print the response: The API sends back a result, which we extract and display.
How It Works Behind the Scenes
Your Python script sends a request to OpenAI’s API.
The API processes your prompt using ChatGPT.
The response is returned in JSON format.
The Python code extracts and prints the relevant data.
Final Thoughts
APIs are essential tools for developers, enabling the connection of different services and automating processes.
With the ChatGPT API, Python developers can easily build chatbots or interactive applications that leverage AI.
The more you practice with APIs, the more creative your projects can become!
Try experimenting with the code above—replace the prompt or tweak the logic to see how different requests yield various responses.
With APIs, the possibilities are endless.
Happy coding! 🚀
Let’s Inspire Future AI Coders Together! ☕
I’m excited to continue sharing my passion for Python programming and AI with you all.
If you’ve enjoyed the content and found it helpful, do consider supporting my work with a small gift.
Just click the link below to make a difference – it’s quick, easy, and every bit helps and motivates me to keep creating awesome contents for you.
Thank you for being amazing!
🎉 We want to hear from you! 🎉 How do you feel about our latest newsletter? Your feedback will help us make it even more awesome! |