- CodeCraft by Dr. Christine Lee
- Posts
- 👉Enhance Career App: Adding Profiles, Data, and AI Inside! 🌟
👉Enhance Career App: Adding Profiles, Data, and AI Inside! 🌟
Powered by Python and PySimpleGUI
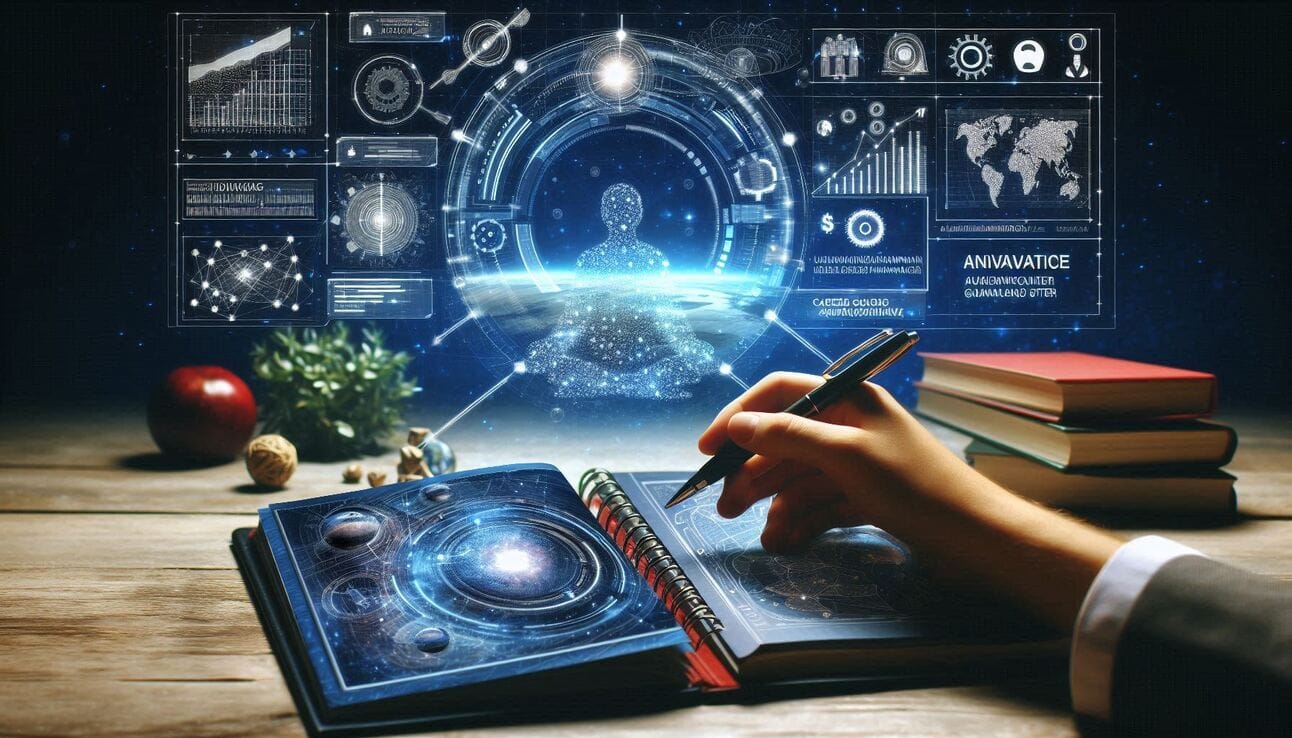
In the previous post, we built a simple yet powerful Career Guidance Advisor app that helps users find the right career paths based on their interests, skills, and goals.
Now, it's time to take things up a notch!
In this post, we’ll explore how to enhance the app by adding features like saving user profiles, incorporating detailed career data, and even using AI for smarter recommendations.
This is going to be fun and educational, especially for beginners!
Let’s get started.
Step 1: Saving User Profiles 📝
The first feature we’ll add is the ability to save user profiles.
This means users can input their preferences—like interests, skills, and goals—save them, and load them later.
This makes the app more personalized and user-friendly.
The Code:
import json
# Sample user profile data
user_profiles = {}
def save_profile(username, profile_data):
user_profiles[username] = profile_data
with open('profiles.json', 'w') as file:
json.dump(user_profiles, file)
print(f"Profile for {username} saved successfully!")
def load_profile(username):
with open('profiles.json', 'r') as file:
profiles = json.load(file)
return profiles.get(username, "Profile not found")
# Example usage
# Profile for John Doe
profile_john = {'interests': ['technology', 'design'], 'skills': ['Python', 'UX/UI'], 'goals': ['Become a software engineer']}
save_profile('john_doe', profile_john)
# Profile for Jane Smith
profile_jane = {'interests': ['science', 'healthcare'], 'skills': ['biology', 'data analysis'], 'goals': ['Pursue a career in data science']}
save_profile('jane_smith', profile_jane)
# Profile for Mark Jones
profile_mark = {'interests': ['art', 'creativity'], 'skills': ['graphic design', 'illustration'], 'goals': ['Work as a graphic designer']}
save_profile('mark_jones', profile_mark)
# Profile for Alice Brown
profile_alice = {'interests': ['finance', 'technology'], 'skills': ['accounting', 'Python'], 'goals': ['Become a financial analyst']}
save_profile('alice_brown', profile_alice)
# Profile for Bob White
profile_bob = {'interests': ['sports', 'nutrition'], 'skills': ['personal training', 'diet planning'], 'goals': ['Become a sports nutritionist']}
save_profile('bob_white', profile_bob)
# Loading and printing a specific profile
loaded_profile = load_profile('jane_smith')
print(loaded_profile)
Explanation:
We start by using a dictionary,
user_profiles
, to store profile data.The
save_profile
function saves a user's profile to a file calledprofiles.json
using Python'sjson
module. This allows us to save and load user data easily.The
load_profile
function retrieves a user’s profile from the JSON file.
This functionality is key to making your app more personalized, as users can now save their data and retrieve it whenever they return to the app.

profiles.json Created
About Python’s JSON Module and File
Python's json
module is a powerful tool for working with JSON (JavaScript Object Notation) data.
It allows you to easily convert Python objects, such as dictionaries and lists, into JSON format and vice versa.
This makes it simple to read from or write to JSON files, which are commonly used for storing configuration settings, data exchange between web services, or even storing user preferences in applications.
By using json
, you can efficiently serialize (convert) Python data into a string that can be saved to a file or transmitted, and deserialize (parse) it back into Python objects when needed.

Content of profiles.json
Step 2: Incorporating Detailed Career Data 🎓💼
Next, let's add more detailed career data.
This could include information like salary ranges, job market trends, educational requirements, and more.
The Code:
careers = {
'Software Engineer': {'salary_range': '80k-120k', 'job_trends': 'Growing', 'education': 'Bachelor\'s Degree in Computer Science'},
'Graphic Designer': {'salary_range': '40k-70k', 'job_trends': 'Stable', 'education': 'Bachelor\'s Degree in Graphic Design'},
'Data Scientist': {'salary_range': '90k-130k', 'job_trends': 'High Demand', 'education': 'Bachelor\'s or Master\'s in Data Science'}
}
def get_career_details(career_name):
return careers.get(career_name, "Career details not found")
# Example usage
career_details = get_career_details('Software Engineer')
print(career_details)
Explanation:
We create a dictionary,
careers
, that holds detailed information about various career paths.The
get_career_details
function retrieves details about a specific career, which can be displayed to the user.
By including more detailed information, your app can provide comprehensive advice, helping users make informed career decisions.

Sample Usage of Detailed Career Data
Step 3: Adding AI for Smarter Recommendations 🤖💡
Finally, let’s sprinkle some AI into the mix.
By integrating a simple AI algorithm, your app can make smarter, more personalised career recommendations.
The Code:
from sklearn.neighbors import KNeighborsClassifier
from sklearn.preprocessing import LabelEncoder
# Sample data: interests, skills, goals mapped to career paths
data = [
['technology', 'coding', 'problem-solving', 'Software Engineer'],
['art', 'creativity', 'design', 'Graphic Designer'],
['math', 'statistics', 'analysis', 'Data Scientist']
]
def train_model(data):
# Extract features and labels
X = [d[:-1] for d in data] # Features: interests, skills, goals
y = [d[-1] for d in data] # Labels: career paths
# Initialize LabelEncoders for each feature column
encoders = [LabelEncoder() for _ in range(len(X[0]))]
# Transform each column in X
X_encoded = []
for col, encoder in zip(zip(*X), encoders):
X_encoded.append(encoder.fit_transform(col))
# Transpose back to original shape
X_encoded = list(zip(*X_encoded))
# Encode the labels
label_encoder = LabelEncoder()
y_encoded = label_encoder.fit_transform(y)
# Train the model
model = KNeighborsClassifier(n_neighbors=1)
model.fit(X_encoded, y_encoded)
# Return the trained model and the encoders
return model, encoders, label_encoder
def predict_career(model, encoders, label_encoder, user_input):
# Encode user input using the same encoders
user_input_encoded = [encoder.transform([feature])[0] for feature, encoder in zip(user_input, encoders)]
predicted_label = model.predict([user_input_encoded])[0]
# Decode the predicted label back to the career path
return label_encoder.inverse_transform([predicted_label])[0]
# Example usage
model, encoders, label_encoder = train_model(data)
user_input = ['technology', 'coding', 'problem-solving']
predicted_career = predict_career(model, encoders, label_encoder, user_input)
print(predicted_career)
Explanation:
We use the
KNeighborsClassifier
from thesklearn
library to create a simple AI model that maps user inputs (interests, skills, goals) to career paths.The
train_model
function trains the model with sample data.Label Encoding: The
LabelEncoder
converts the categorical string data into numeric data. Each unique string is assigned a unique integer.Fitting and Transforming: The features (
X
) and labels (y
) are encoded using thefit_transform()
method, which both fits the encoder to the data and transforms it.Prediction: For prediction, the user input is also transformed using the same encoders that were used to train the model.
The
predict_career
function uses the trained model to predict the best career for a user based on their inputs.
With AI, your app becomes even more powerful, providing users with smarter, data-driven recommendations.

Predicted Career!
Wrapping Up 🎉
In this post, you learned how to enhance your Career Guidance Advisor app by adding user profiles, incorporating detailed career data, and integrating AI for smarter recommendations.
These features not only make the app more useful but also more engaging and personalized for users.
Coding with a Smile 🤣 😂
Type Error Tantrums:
Type errors are like trying to fit a square peg into a round hole.
Python’s there to remind you, “Hey, that’s not going to work. Let’s try something else.”
Recommended Resources 📚
Let’s Inspire Future AI Coders Together! ☕
I’m excited to continue sharing my passion for Python programming and AI with you all. If you’ve enjoyed the content and found it helpful, do consider supporting my work with a small gift. Just click the link below to make a difference – it’s quick, easy, and every bit helps and motivates me to keep creating awesome contents for you.
Thank you for being amazing!
What’s Next? 📅
In the next post, we'll dive deeper into the AI example on KNeighborsClassifier breaking it down step by step to make it easy to understand.
We'll cover how the code uses machine learning techniques to predict career paths based on user input, focusing on how categorical data is converted to numerical form and how the K-Nearest Neighbors algorithm works in this context.
Whether you're new to AI or looking to strengthen your skills, this post will guide you through the process, ensuring you grasp the key concepts and feel confident applying them in your own projects.
Ready for More Python Fun? 📬
Subscribe to our newsletter now and get a free Python cheat sheet! 📑 Dive deeper into Python programming with more exciting projects and tutorials designed just for beginners.
Keep learning, keep coding 👩💻👨💻, and keep discovering new possibilities! 💻✨
Enjoy your journey into artificial intelligence, machine learning, data analytics, data science and more with Python!
Stay tuned for our next exciting project in the following edition!
Happy coding!🚀📊✨
🎉 We want to hear from you! 🎉 How do you feel about our latest newsletter? Your feedback will help us make it even more awesome! |