- CodeCraft by Dr. Christine Lee
- Posts
- Exploring Fun String Methods in Python
Exploring Fun String Methods in Python

Exploring Python string methods
Welcome to our journey into Python strings, where we'll get to know some handy string methods that make working with text not only easy but also fun. These methods allow us to change text, check its content, and do much more with just a few lines of code. Let's dive in with some simple examples to show what each method can do!
The Art of Transformation
Title Case with title()
The title()
method capitalizes the first letter of every word in a string, making it perfect for titles or headings.
book_title = "harry potter and the chamber of secrets"
# Outputs 'Harry Potter And The Chamber Of Secrets'
print(book_title.title())

Fun Fact: Use title()
to create a book title generator for your next bestseller!
Making the First Letter Big with capitalize()
The capitalize()
method is used to convert the first character of a string to uppercase (capital letter) and change all other characters to lowercase. This function is perfect for fixing the capitalization in sentences or names.
name = "john doe"
print(name.capitalize()) # Outputs 'John doe'
In this example, capitalize()
transforms the first letter 'j' of "john doe" into an uppercase 'J', while the rest of the letters remain in lowercase, resulting in "John doe".
Fun Example: Imagine creating a program that automatically corrects the capitalization of names in a guest list for an event. Using capitalize()
, you can ensure each name starts with a capital letter, making the list look polished and professional.
Swap Cases with swapcase()
swapcase()
converts uppercase letters to lowercase and vice versa, adding a playful twist to any text.
message = "PyThOn Is FuN!"
print(message.swapcase()) # Outputs 'pYtHoN iS fUn!'
Fun Game: Create a secret code generator that uses swapcase()
to confuse the readers!
The Analytical Side
Checking Case with islower()
and isupper()
islower()
returns True
if all characters in the string are lowercase, while isupper()
checks for uppercase.
greeting = "hello world"
shout = "HELLO WORLD"
print(greeting.islower()) # Outputs True
print(shout.isupper()) # Outputs True
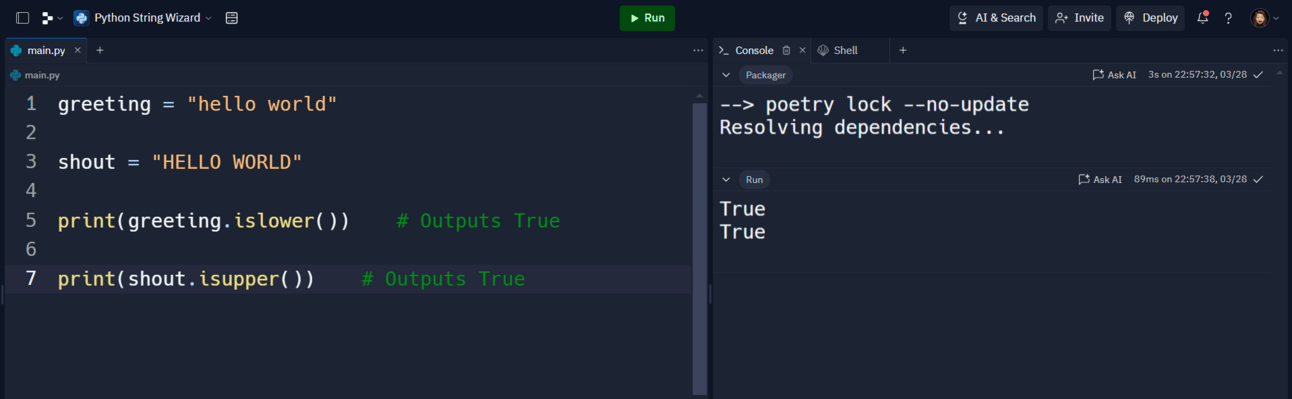
Quiz Idea: Design a case-identifying game where players guess whether a string is all upper or lower case.
Numeric Check with isnumeric()
isnumeric()
determines if all characters in a string are numeric, useful for validating numeric input.
year = "2024"
print(year.isnumeric()) # Outputs True
Fun Activity: Create a number guessing game using isnumeric()
to ensure valid numerical entries.
Whitespace and Alignment
Detecting Spaces with isspace()
The isspace()
method checks if the string contains only whitespace characters (space, tab, newline, etc.).
space = " "
print(space.isspace()) # Outputs True
Fun Use: In a text editor program, use isspace()
to detect and highlight unnecessary whitespace.
Prefixes and Suffixes
Starts With Using startswith()
startswith()
checks if the string begins with the specified prefix, returning True
or False
.
sentence = "Python is awesome"
print(sentence.startswith("Python")) # Outputs True
Game Idea: Build a word matching game where players find words that start with a given prefix.
Exploring these string methods in Python not only enhances your coding toolkit but also opens up creative possibilities for problem-solving and game development. Have fun experimenting with these methods, and watch as they transform ordinary strings into extraordinary data!
Ready to Master Python Strings?
Subscribe to our newsletter for more exciting insights into Python programming. Dive into the world of coding with us, where every string method unlocks new potentials and every line of code tells a story.
🌟 Subscribe and Elevate Your Coding Journey! 🌟
Join us on this adventurous journey through the realms of Python strings, where fun meets function in the world of programming!