- CodeCraft by Dr. Christine Lee
- Posts
- Jumpstart Your AI Journey with Python Libraries! ๐๐ค
Jumpstart Your AI Journey with Python Libraries! ๐๐ค

Introduction
Hello, future AI enthusiasts! ๐
Are you ready to dive into the world of Artificial Intelligence (AI) but not sure where to start? No worries, weโve got you covered!
Today, weโll embark on an exciting journey to explore how you can use Python libraries to develop amazing AI applications.
Letโs get our hands dirty with some code and have fun along the way! ๐
Why Python for AI?
Python is like the magical Swiss Army knife of programming languagesโit's versatile, easy to learn, and packed with powerful libraries that make developing AI applications a breeze. Hereโs why Python is perfect for beginners in AI:
Simple Syntax: Pythonโs syntax is clean and readable, making it easy to pick up for beginners.
Huge Community: Thereโs a massive community of Python developers who are always ready to help.
Powerful Libraries: Python comes with a variety of libraries that can handle everything from data manipulation to building complex neural networks.
Letโs Meet Some Python Libraries! ๐๐
1. NumPy: The Math Wizard ๐งโโ๏ธ
What It Does:
NumPy is the go-to library for numerical computations. It can handle large multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays.
Example:
Want to add two arrays? NumPy makes it super easy!
import numpy as np
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
result = array1 + array2
print(result) # Output: [5 7 9]
Explanation:
import numpy as np
: This imports the NumPy library and gives it the alias np for convenience.array1 = np.array([1, 2, 3]) and array2 = np.array([4, 5, 6])
: These lines create two NumPy arrays.result = array1 + array2
: This adds the two arrays element-wise.print(result):
This prints the resulting array [5 7 9].

2. Pandas: The Data Guru ๐
What It Does:
Pandas is your best friend when it comes to data manipulation and analysis. It provides data structures like DataFrames, which make it easy to handle and analyze data.
Example:
Reading a CSV file is a breeze with Pandas!
import pandas as pd
data = pd.read_csv('data.csv')
print(data.head())
Explanation:
import pandas as pd
: This imports the Pandas library and gives it the alias pd.data = pd.read_csv('data.csv')
: This reads a CSV file named 'data.csv' into a DataFrame.print(data.head())
: This prints the first five rows of the DataFrame to give you a quick look at the data.
3. Matplotlib: The Chart Artist ๐จ
What It Does:
Matplotlib is a plotting library that helps you create static, animated, and interactive visualizations in Python.
Example:
Creating a simple line plot.
import matplotlib.pyplot as plt
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
plt.plot(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Simple Line Plot')
plt.show()
Explanation:
import matplotlib.pyplot as plt: This imports the plotting library Matplotlib.
x = [1, 2, 3, 4, 5] and y = [2, 3, 5, 7, 11]: These define the data points for the x-axis and y-axis.
plt.plot(x, y): This creates a line plot with x and y data.
plt.xlabel('X-axis') and plt.ylabel('Y-axis'): These label the x-axis and y-axis.
plt.title('Simple Line Plot'): This adds a title to the plot.
plt.show(): This displays the plot.

4. Scikit-Learn: The Machine Learning Pro ๐
What It Does:
Scikit-Learn is a machine learning library that provides simple and efficient tools for data mining and data analysis. Itโs built on NumPy, SciPy, and Matplotlib.
Example:
Training a simple model to predict house prices.
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
# Sample data
X = [[1], [2], [3], [4], [5]]
y = [2, 3, 5, 7, 11]
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train the model
model = LinearRegression()
model.fit(X_train, y_train)
# Predict
predictions = model.predict(X_test)
print(predictions)
Explanation:
from sklearn.model_selection import train_test_split and from sklearn.linear_model import LinearRegression
: These lines import functions and classes from Scikit-Learn.X = [[1], [2], [3], [4], [5]] and y = [2, 3, 5, 7, 11]
: These define the features and target variable for the model.X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
: This splits the data into training and testing sets.model = LinearRegression()
: This creates an instance of the Linear Regression model.model.fit(X_train, y_train)
: This trains the model using the training data.predictions = model.predict(X_test)
: This makes predictions on the test data.print(predictions)
: This prints the predictions made by the model.
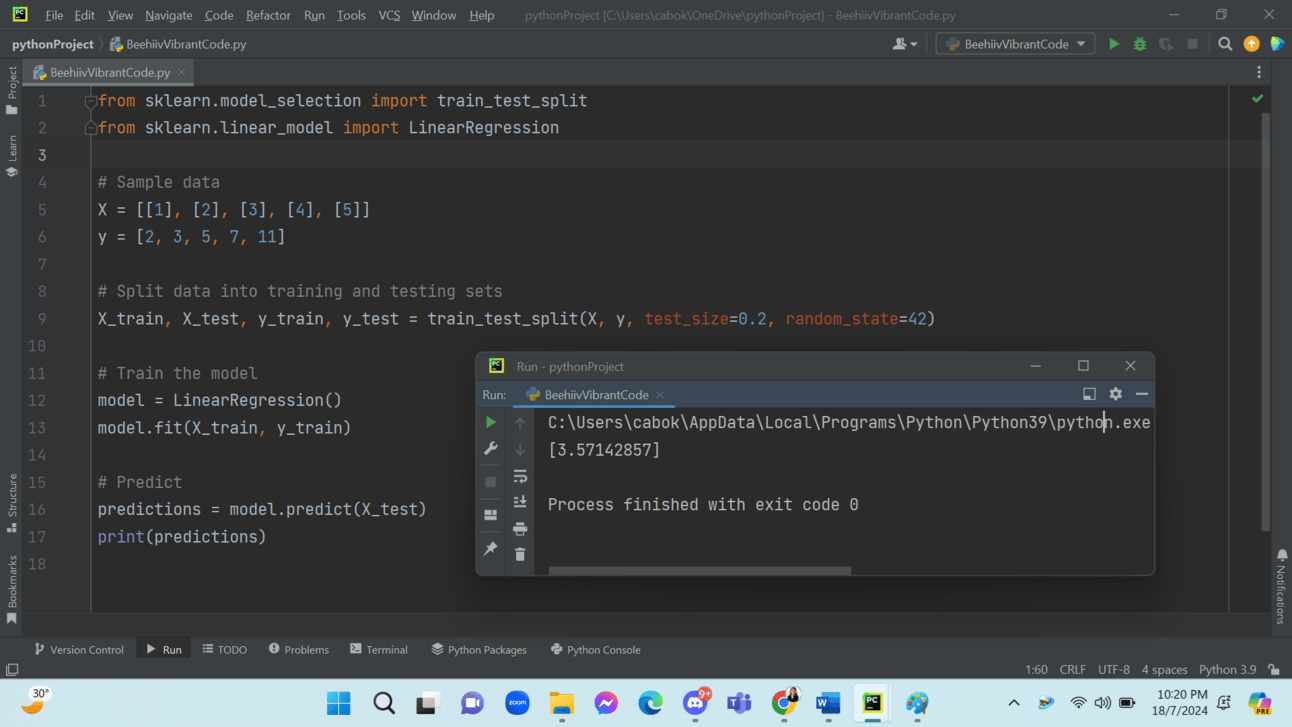
5. TensorFlow: The Deep Learning Powerhouse ๐ง
What It Does:
TensorFlow is a powerful open-source library for numerical computation and machine learning. Itโs particularly good for building and training deep learning models.
Example:
Building a simple neural network.
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
# Create a simple model
model = Sequential([
Dense(10, input_shape=(1,), activation='relu'),
Dense(1, activation='linear')
])
# Compile the model
model.compile(optimizer='adam', loss='mean_squared_error')
# Summary of the model
model.summary()
Explanation:
import tensorflow as tf and from tensorflow.keras.models import Sequential and from tensorflow.keras.layers import Dense
: These lines import TensorFlow and its Keras API for building neural networks.model = Sequential([...])
: This creates a Sequential model, which is a linear stack of layers.Dense(10, input_shape=(1,), activation='relu')
: This adds a dense (fully connected) layer with 10 neurons and ReLU activation function. The input shape is set to 1.Dense(1, activation='linear')
: This adds another dense layer with 1 neuron and a linear activation function.model.compile(optimizer='adam', loss='mean_squared_error')
: This compiles the model with Adam optimizer and mean squared error loss function.model.summary()
: This prints a summary of the model architecture.

Summary
Congratulations! ๐ Youโve just taken your first steps into the world of AI using Python libraries. Weโve introduced you to some of the most powerful tools in the AI toolbox, from NumPyโs numerical prowess to TensorFlowโs deep learning capabilities. With these libraries, you can start building your own AI applications and unlock a world of possibilities!
Coding with a Smile๐คฃ๐
Debugger's Best Friend:
The feeling of finally understanding how to use a debugger is akin to finding a flashlight in a dark cave. Youโre still in the cave, but at least now you can see all the bats (bugs) you need to deal with!
Recommended Resources ๐
Subscribe to Unsupervised Learning for a security-focused AI builder's unique insights, tools, and strategies on crafting a successful and meaningful life in the AI era.
Ready for More Python Fun? ๐ฌ
Subscribe to our newsletter now and get a free Python cheat sheet! ๐ Dive deeper into Python programming with more exciting projects and tutorials designed just for beginners.
Keep learning, keep coding ๐ฉโ๐ป๐จโ๐ป, and keep discovering new possibilities! ๐ปโจ
Enjoy your journey into artificial intelligence, machine learning, data analytics, data science and more with Python!
Stay tuned for our next exciting project in the following edition!
Happy coding!๐๐โจ
๐ We want to hear from you! ๐ How do you feel about our latest newsletter? Your feedback will help us make it even more awesome! |